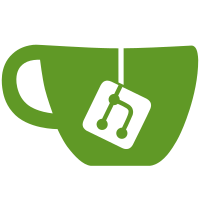
methods and reimplement computeBound so that it passes back a bounding volume rather than modifying the local one.
145 lines
5.9 KiB
C++
145 lines
5.9 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2005 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSG_LOD
|
|
#define OSG_LOD 1
|
|
|
|
#include <osg/Group>
|
|
|
|
namespace osg {
|
|
|
|
/** LOD - Level Of Detail group node which allows switching between children
|
|
depending on distance from eye point.
|
|
Typical uses are for load balancing - objects further away from
|
|
the eye point are rendered at a lower level of detail, and at times
|
|
of high stress on the graphics pipeline lower levels of detail can
|
|
also be chosen.
|
|
The children are ordered from most detailed (for close up views) to the least
|
|
(see from a distance), and a set of ranges are used to decide which LOD is used
|
|
at different view distances, the criteria used is child 'i' is used when
|
|
range[i]<dist<range[i+1] is true. This requires there to be n+1 range values where the number of
|
|
children is n, since no maximum distance of infinity is assumed. If the number of range values (m)
|
|
is insufficient then the children m through to n will be ignored, only 0..m-1 will be used
|
|
during rendering.
|
|
*/
|
|
class OSG_EXPORT LOD : public Group
|
|
{
|
|
public :
|
|
|
|
LOD();
|
|
|
|
/** Copy constructor using CopyOp to manage deep vs shallow copy.*/
|
|
LOD(const LOD&,const CopyOp& copyop=CopyOp::SHALLOW_COPY);
|
|
|
|
META_Node(osg, LOD);
|
|
|
|
virtual void traverse(NodeVisitor& nv);
|
|
|
|
virtual bool addChild(Node *child);
|
|
|
|
virtual bool addChild(Node *child, float min, float max);
|
|
|
|
virtual bool removeChild(Node *child);
|
|
|
|
|
|
typedef std::pair<float,float> MinMaxPair;
|
|
typedef std::vector<MinMaxPair> RangeList;
|
|
|
|
/** Modes which control how the center of object should be determined when computed which child is active.*/
|
|
enum CenterMode
|
|
{
|
|
USE_BOUNDING_SPHERE_CENTER,
|
|
USER_DEFINED_CENTER
|
|
};
|
|
|
|
/** Set how the center of object should be determined when computed which child is active.*/
|
|
void setCenterMode(CenterMode mode) { _centerMode=mode; }
|
|
|
|
/** Get how the center of object should be determined when computed which child is active.*/
|
|
CenterMode getCenterMode() const { return _centerMode; }
|
|
|
|
/** Sets the object-space point which defines the center of the osg::LOD.
|
|
center is affected by any transforms in the hierarchy above the osg::LOD.*/
|
|
inline void setCenter(const Vec3& center) { _centerMode=USER_DEFINED_CENTER; _userDefinedCenter = center; }
|
|
|
|
/** return the LOD center point. */
|
|
inline const Vec3& getCenter() const { if (_centerMode==USER_DEFINED_CENTER) return _userDefinedCenter; else return getBound().center(); }
|
|
|
|
|
|
/** Set the object-space reference radius of the volume enclosed by the LOD.
|
|
* Used to detmine the bounding sphere of the LOD in the absense of any children.*/
|
|
inline void setRadius(float radius) { _radius = radius; }
|
|
|
|
/** Get the object-space radius of the volume enclosed by the LOD.*/
|
|
inline float getRadius() const { return _radius; }
|
|
|
|
|
|
|
|
/** Modes that control how the range values should be intepreted when computing which child is active.*/
|
|
enum RangeMode
|
|
{
|
|
DISTANCE_FROM_EYE_POINT,
|
|
PIXEL_SIZE_ON_SCREEN
|
|
};
|
|
|
|
/** Set how the range values should be intepreted when computing which child is active.*/
|
|
void setRangeMode(RangeMode mode) { _rangeMode = mode; }
|
|
|
|
/** Get how the range values should be intepreted when computing which child is active.*/
|
|
RangeMode getRangeMode() const { return _rangeMode; }
|
|
|
|
|
|
/** Sets the min and max visible ranges of range of specifiec child.
|
|
Values are floating point distance specified in local objects coordinates.*/
|
|
void setRange(unsigned int childNo, float min,float max);
|
|
|
|
/** returns the min visible range for specified child.*/
|
|
inline float getMinRange(unsigned int childNo) const { return _rangeList[childNo].first; }
|
|
|
|
/** returns the max visible range for specified child.*/
|
|
inline float getMaxRange(unsigned int childNo) const { return _rangeList[childNo].second; }
|
|
|
|
/** returns the number of ranges currently set.
|
|
* An LOD which has been fully set up will have getNumChildren()==getNumRanges(). */
|
|
inline unsigned int getNumRanges() const { return _rangeList.size(); }
|
|
|
|
/** set the list of MinMax ranges for each child.*/
|
|
inline void setRangeList(const RangeList& rangeList) { _rangeList=rangeList; }
|
|
|
|
/** return the list of MinMax ranges for each child.*/
|
|
inline const RangeList& getRangeList() const { return _rangeList; }
|
|
|
|
virtual BoundingSphere computeBound() const;
|
|
|
|
protected :
|
|
virtual ~LOD() {}
|
|
|
|
virtual void childRemoved(unsigned int pos, unsigned int numChildrenToRemove);
|
|
virtual void childInserted(unsigned int pos);
|
|
|
|
virtual void rangeRemoved(unsigned int /*pos*/, unsigned int /*numChildrenToRemove*/) {}
|
|
virtual void rangeInserted(unsigned int /*pos*/) {}
|
|
|
|
CenterMode _centerMode;
|
|
Vec3 _userDefinedCenter;
|
|
float _radius;
|
|
|
|
RangeMode _rangeMode;
|
|
RangeList _rangeList;
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|