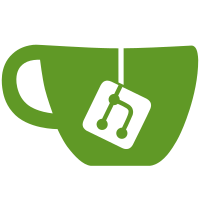
Very simple cases of state configuration are supported (all the ones I really need): - single per pixel not attenuated non spot light source ON/OFF - exp2 fog ON/OFF - diffuse texture in rgb + optional specular gloss in alpha (Texture unit 0) ON/OFF - normal map texture (Texture unit 1 and Tangent in VertexAttribArray 6) ON/OFF - blending and alpha testing (not in shader pipeline) To view fixed function pipeline files and paged databases simply run >osgshadergen myfile.osg"
85 lines
2.3 KiB
C++
85 lines
2.3 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2009 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
/**
|
|
* \brief Shader generator framework.
|
|
* \author Maciej Krol
|
|
*/
|
|
|
|
#ifndef OSGUTIL_SHADER_STATE_
|
|
#define OSGUTIL_SHADER_STATE_ 1
|
|
|
|
#include <osgUtil/Export>
|
|
#include <osg/NodeVisitor>
|
|
#include <osg/State>
|
|
|
|
namespace osgUtil
|
|
{
|
|
|
|
class OSGUTIL_EXPORT ShaderGenCache : public osg::Referenced
|
|
{
|
|
public:
|
|
enum StateMask
|
|
{
|
|
BLEND = 1,
|
|
LIGHTING = 2,
|
|
FOG = 4,
|
|
DIFFUSE_MAP = 8, //< Texture in unit 0
|
|
NORMAL_MAP = 16 //< Texture in unit 1 and vertex attribute array 6
|
|
};
|
|
|
|
typedef std::map<unsigned int, osg::ref_ptr<osg::StateSet> > StateSetMap;
|
|
|
|
ShaderGenCache() {};
|
|
|
|
void setStateSet(unsigned int stateMask, osg::StateSet *program);
|
|
osg::StateSet *getStateSet(unsigned int stateMask) const;
|
|
osg::StateSet *getOrCreateStateSet(unsigned int stateMask);
|
|
|
|
protected:
|
|
osg::StateSet *createStateSet(unsigned int stateMask) const;
|
|
mutable OpenThreads::Mutex _mutex;
|
|
StateSetMap _stateSetMap;
|
|
|
|
};
|
|
|
|
class OSGUTIL_EXPORT ShaderGenVisitor : public osg::NodeVisitor
|
|
{
|
|
public:
|
|
ShaderGenVisitor();
|
|
ShaderGenVisitor(ShaderGenCache *stateCache);
|
|
|
|
void setStateCache(ShaderGenCache *stateCache) { _stateCache = stateCache; }
|
|
ShaderGenCache *getStateCache() const { return _stateCache.get(); }
|
|
|
|
/// Top level state set applied as the first one.
|
|
void setRootStateSet(osg::StateSet *stateSet);
|
|
osg::StateSet *getRootStateSet() const { return _rootStateSet.get(); }
|
|
|
|
void apply(osg::Node &node);
|
|
void apply(osg::Geode &geode);
|
|
|
|
void reset();
|
|
|
|
protected:
|
|
void update(osg::Drawable *drawable);
|
|
|
|
osg::ref_ptr<ShaderGenCache> _stateCache;
|
|
osg::ref_ptr<osg::State> _state;
|
|
osg::ref_ptr<osg::StateSet> _rootStateSet;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|