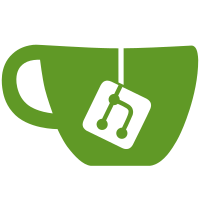
for settings options in osgDB::Registry, and added the paramter to all of the reaader/writer plugins. The Options structure by default has an string attached for packing basic options, however, it also can be subclassed to encapsulate any users defined option data. In the later case both the client code *and* the plugin need to be aware of subclass, the plugin will need to use dynamic_cast<> to assertain its type.
57 lines
1.8 KiB
Plaintext
57 lines
1.8 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2001 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSGDB_READERWRITER
|
|
#define OSGDB_READERWRITER 1
|
|
|
|
#include <osg/Referenced>
|
|
#include <osg/Image>
|
|
#include <osg/Node>
|
|
|
|
#include <string>
|
|
|
|
namespace osgDB {
|
|
|
|
|
|
/** pure virtual base class for reading and writing of non native formats. */
|
|
class OSGDB_EXPORT ReaderWriter : public osg::Referenced
|
|
{
|
|
public:
|
|
virtual ~ReaderWriter() {}
|
|
virtual const char* className() = 0;
|
|
virtual bool acceptsExtension(const std::string& /*extension*/) { return false; }
|
|
|
|
class Options : public osg::Referenced
|
|
{
|
|
public:
|
|
|
|
Options() {}
|
|
Options(const std::string& str):_str(str) {}
|
|
|
|
void setOptionString(const std::string& str) { _str = str; }
|
|
const std::string& getOptionString() const { return _str; }
|
|
|
|
protected:
|
|
|
|
virtual ~Options() {}
|
|
|
|
std::string _str;
|
|
|
|
};
|
|
|
|
|
|
virtual osg::Object* readObject(const std::string& /*fileName*/,const Options* =NULL) { return NULL; }
|
|
virtual osg::Image* readImage(const std::string& /*fileName*/,const Options* =NULL) { return NULL; }
|
|
virtual osg::Node* readNode(const std::string& /*fileName*/,const Options* =NULL) { return NULL; }
|
|
|
|
virtual bool writeObject(const osg::Object& /*obj*/,const std::string& /*fileName*/,const Options* =NULL) {return false; }
|
|
virtual bool writeImage(const osg::Image& /*image*/,const std::string& /*fileName*/,const Options* =NULL) {return false; }
|
|
virtual bool writeNode(const osg::Node& /*node*/,const std::string& /*fileName*/,const Options* =NULL) { return false; }
|
|
};
|
|
|
|
|
|
};
|
|
|
|
#endif // OSG_READERWRITER
|