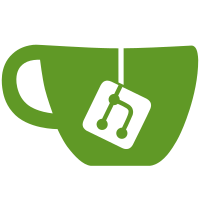
//C++ header to help scripts and editors pick up the fact that the file is a header file.
75 lines
2.3 KiB
Plaintext
75 lines
2.3 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2001 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSGUTIL_TRACKBALLMANIPULATOR
|
|
#define OSGUTIL_TRACKBALLMANIPULATOR 1
|
|
|
|
#include <osgUtil/CameraManipulator>
|
|
|
|
namespace osgUtil{
|
|
|
|
class OSGUTIL_EXPORT TrackballManipulator : public CameraManipulator
|
|
{
|
|
public:
|
|
|
|
TrackballManipulator();
|
|
virtual ~TrackballManipulator();
|
|
|
|
/** Attach a node to the manipulator.
|
|
Automatically detaches previously attached node.
|
|
setNode(NULL) detaches previously nodes.
|
|
Is ignored by manipulators which do not require a reference model.*/
|
|
virtual void setNode(osg::Node*);
|
|
|
|
/** Return node if attached.*/
|
|
virtual const osg::Node* getNode() const;
|
|
|
|
/** Move the camera to the default position.
|
|
May be ignored by manipulators if home functionality is not appropriate.*/
|
|
virtual void home(const GUIEventAdapter& ea,GUIActionAdapter& us);
|
|
|
|
/** Start/restart the manipulator.*/
|
|
virtual void init(const GUIEventAdapter& ea,GUIActionAdapter& us);
|
|
|
|
|
|
/** handle events, return true if handled, false otherwise.*/
|
|
virtual bool handle(const GUIEventAdapter& ea,GUIActionAdapter& us);
|
|
|
|
private:
|
|
|
|
/** Reset the internal GUIEvent stack.*/
|
|
void flushMouseEventStack();
|
|
/** Add the current mouse GUIEvent to internal stack.*/
|
|
void addMouseEvent(const GUIEventAdapter& ea);
|
|
|
|
/** For the give mouse movement calculate the movement of the camera.
|
|
Return true is camera has moved and a redraw is required.*/
|
|
bool calcMovement();
|
|
|
|
void trackball(osg::Vec3& axis,float& angle, float p1x, float p1y, float p2x, float p2y);
|
|
float tb_project_to_sphere(float r, float x, float y);
|
|
|
|
|
|
/** Check the speed at which the mouse is moving.
|
|
If speed is below a threshold then return false, otherwise return true.*/
|
|
bool isMouseMoving();
|
|
|
|
// Internal event stack comprising last three mouse events.
|
|
osg::ref_ptr<const GUIEventAdapter> _ga_t1;
|
|
osg::ref_ptr<const GUIEventAdapter> _ga_t0;
|
|
|
|
osg::ref_ptr<osg::Node> _node;
|
|
|
|
float _modelScale;
|
|
float _minimumZoomScale;
|
|
|
|
bool _thrown;
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|
|
|