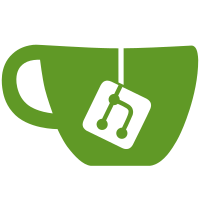
and passed as paramters into straight forward non const built in types, i.e. const bool foogbar(const int) becomes bool foobar(int).
53 lines
1.6 KiB
Plaintext
53 lines
1.6 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2002 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSG_OCCLUDERNODE
|
|
#define OSG_OCCLUDERNODE 1
|
|
|
|
#include <osg/Group>
|
|
#include <osg/ConvexPlanarOccluder>
|
|
|
|
namespace osg {
|
|
|
|
/** OccluderNode is a Group node which allows OccluderNodeing between children.
|
|
Typical uses would be for objects which might need to be rendered
|
|
differently at different times, for instance a OccluderNode could be used
|
|
to represent the different states of a traffic light.
|
|
*/
|
|
class SG_EXPORT OccluderNode : public Group
|
|
{
|
|
public :
|
|
|
|
OccluderNode();
|
|
|
|
/** Copy constructor using CopyOp to manage deep vs shallow copy.*/
|
|
OccluderNode(const OccluderNode&,const CopyOp& copyop=CopyOp::SHALLOW_COPY);
|
|
|
|
META_Node(osg, OccluderNode);
|
|
|
|
|
|
/** Attach a ConvexPlanarOccluder to an OccluderNode.*/
|
|
void setOccluder(ConvexPlanarOccluder* occluder) { _occluder = occluder; }
|
|
|
|
/** Get the ConvexPlanarOccluder* attached to a OccluderNode. */
|
|
ConvexPlanarOccluder* getOccluder() { return _occluder.get(); }
|
|
|
|
/** Get the const ConvexPlanarOccluder* attached to a OccluderNode.*/
|
|
const ConvexPlanarOccluder* getOccluder() const { return _occluder.get(); }
|
|
|
|
|
|
protected :
|
|
|
|
virtual ~OccluderNode() {}
|
|
|
|
/** Override's Group's computeBound.*/
|
|
virtual bool computeBound() const;
|
|
|
|
ref_ptr<ConvexPlanarOccluder> _occluder;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|