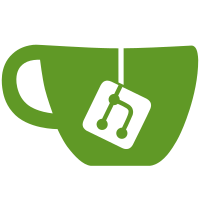
and passed as paramters into straight forward non const built in types, i.e. const bool foogbar(const int) becomes bool foobar(int).
62 lines
1.8 KiB
Plaintext
62 lines
1.8 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2002 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSGUTIL_INSERTIMPOSTORSVISITOR
|
|
#define OSGUTIL_INSERTIMPOSTORSVISITOR
|
|
|
|
#include <osg/NodeVisitor>
|
|
#include <osg/Impostor>
|
|
|
|
#include <osgUtil/Export>
|
|
|
|
namespace osgUtil {
|
|
|
|
/** Insert impostor nodes into scene graph.
|
|
* For example of usage see src/Demos/osgimpostor.
|
|
*/
|
|
class OSGUTIL_EXPORT InsertImpostorsVisitor : public osg::NodeVisitor
|
|
{
|
|
public:
|
|
|
|
/// default to traversing all children.
|
|
InsertImpostorsVisitor();
|
|
|
|
void setImpostorThresholdRatio(float ratio) { _impostorThresholdRatio = ratio; }
|
|
float getImpostorThresholdRatio() const { return _impostorThresholdRatio; }
|
|
|
|
void setMaximumNumberOfNestedImpostors(unsigned int num) { _maximumNumNestedImpostors = num; }
|
|
unsigned int getMaximumNumberOfNestedImpostors() const { return _maximumNumNestedImpostors; }
|
|
|
|
/** empty visitor, make it ready for next traversal.*/
|
|
void reset();
|
|
|
|
virtual void apply(osg::Node& node);
|
|
|
|
virtual void apply(osg::Group& node);
|
|
|
|
virtual void apply(osg::LOD& node);
|
|
|
|
virtual void apply(osg::Impostor& node);
|
|
|
|
/* insert the required impostors into the scene graph.*/
|
|
void insertImpostors();
|
|
|
|
protected:
|
|
|
|
typedef std::vector< osg::Group* > GroupList;
|
|
typedef std::vector< osg::LOD* > LODList;
|
|
|
|
GroupList _groupList;
|
|
LODList _lodList;
|
|
|
|
float _impostorThresholdRatio;
|
|
unsigned int _maximumNumNestedImpostors;
|
|
unsigned int _numNestedImpostors;
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|