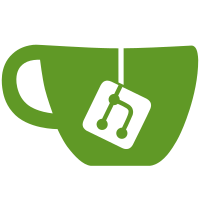
in progress for the new support for controlling the projection matrix from within the scene graph. Also Added osg::State::getClippingVolume(), getProjectionMatrix() and getModelViewMatrix() with allows drawables to access the current projection, and model view matrices and the view frustum in local coords to the drawable.
87 lines
2.1 KiB
Plaintext
87 lines
2.1 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2001 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSGUTIL_RENDERLEAF
|
|
#define OSGUTIL_RENDERLEAF 1
|
|
|
|
#include <osg/ref_ptr>
|
|
#include <osg/Matrix>
|
|
#include <osg/Drawable>
|
|
#include <osg/State>
|
|
|
|
#include <osgUtil/Export>
|
|
|
|
namespace osgUtil {
|
|
|
|
// forward declare RenderGraph
|
|
class RenderGraph;
|
|
|
|
/** container class for all data required for rendering of drawables.
|
|
*/
|
|
class OSGUTIL_EXPORT RenderLeaf : public osg::Referenced
|
|
{
|
|
public:
|
|
|
|
|
|
inline RenderLeaf(osg::Drawable* drawable,osg::Matrix* projection,osg::Matrix* modelview, float depth=0.0f):
|
|
_parent(NULL),
|
|
_drawable(drawable),
|
|
_projection(projection),
|
|
_modelview(modelview),
|
|
_depth(depth) {}
|
|
|
|
|
|
inline void set(osg::Drawable* drawable,osg::Matrix* projection,osg::Matrix* modelview, float depth=0.0f)
|
|
{
|
|
_parent = NULL;
|
|
_drawable = drawable;
|
|
_projection = projection,
|
|
_modelview = modelview,
|
|
_depth = depth;
|
|
}
|
|
|
|
inline void reset()
|
|
{
|
|
_parent = NULL;
|
|
_drawable = NULL;
|
|
_projection = NULL;
|
|
_modelview = NULL;
|
|
_depth = 0.0f;
|
|
}
|
|
|
|
|
|
virtual void render(osg::State& state,RenderLeaf* previous);
|
|
|
|
/// allow RenderGraph to change the RenderLeaf's _parent.
|
|
friend class osgUtil::RenderGraph;
|
|
|
|
public:
|
|
|
|
RenderGraph* _parent;
|
|
osg::Drawable* _drawable;
|
|
osg::ref_ptr<osg::Matrix> _projection;
|
|
osg::ref_ptr<osg::Matrix> _modelview;
|
|
float _depth;
|
|
|
|
private:
|
|
|
|
/// disallow creation of blank RenderLeaf as this isn't useful.
|
|
RenderLeaf():
|
|
_parent(NULL),
|
|
_drawable(NULL),
|
|
_projection(NULL),
|
|
_modelview(NULL),
|
|
_depth(0.0f) {}
|
|
|
|
/// disallow copy construction.
|
|
RenderLeaf(const RenderLeaf&):osg::Referenced() {}
|
|
/// disallow copy operator.
|
|
RenderLeaf& operator = (const RenderLeaf&) { return *this; }
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|