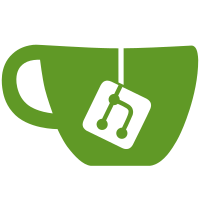
the text implementation to provide better speed, especially by using the alignement to screen option. Deprecated the Text::setFontSize(,) method, which is now being replaced by setFontResolution(,) Fixed typos in Texture*.cpp. Removed old deprecated methods from osg headers.
147 lines
5.3 KiB
C++
147 lines
5.3 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2003 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSG_TEXTURE2D
|
|
#define OSG_TEXTURE2D 1
|
|
|
|
#include <osg/Texture>
|
|
|
|
namespace osg {
|
|
|
|
/** Texture state class which encapsulates OpenGl texture functionality.*/
|
|
class SG_EXPORT Texture2D : public Texture
|
|
{
|
|
|
|
public :
|
|
|
|
Texture2D();
|
|
|
|
/** Copy constructor using CopyOp to manage deep vs shallow copy.*/
|
|
Texture2D(const Texture2D& text,const CopyOp& copyop=CopyOp::SHALLOW_COPY);
|
|
|
|
META_StateAttribute(osg, Texture2D,TEXTURE);
|
|
|
|
/** return -1 if *this < *rhs, 0 if *this==*rhs, 1 if *this>*rhs.*/
|
|
virtual int compare(const StateAttribute& rhs) const;
|
|
|
|
virtual void getAssociatedModes(std::vector<GLMode>& modes) const
|
|
{
|
|
modes.push_back(GL_TEXTURE_2D);
|
|
}
|
|
|
|
/** Set the texture image. */
|
|
void setImage(Image* image);
|
|
|
|
/** Get the texture image. */
|
|
Image* getImage() { return _image.get(); }
|
|
|
|
/** Get the const texture image. */
|
|
inline const Image* getImage() const { return _image.get(); }
|
|
|
|
inline unsigned int& getModifiedTag(unsigned int contextID) const
|
|
{
|
|
// get the modified tag for the current contextID.
|
|
return _modifiedTag[contextID];
|
|
}
|
|
|
|
/** Set the texture width and height. If width or height are zero then
|
|
* the repsective size value is calculated from the source image sizes. */
|
|
inline void setTextureSize(int width, int height) const
|
|
{
|
|
_textureWidth = width;
|
|
_textureHeight = height;
|
|
}
|
|
|
|
int getTextureWidth() const { return _textureWidth; }
|
|
int getTextureHeight() const { return _textureHeight; }
|
|
|
|
// deprecated.
|
|
inline void getTextureSize(int& width, int& height) const
|
|
{
|
|
width = _textureWidth;
|
|
height = _textureHeight;
|
|
}
|
|
|
|
|
|
class SG_EXPORT SubloadCallback : public Referenced
|
|
{
|
|
public:
|
|
virtual void load(const Texture2D& texture,State& state) const = 0;
|
|
virtual void subload(const Texture2D& texture,State& state) const = 0;
|
|
};
|
|
|
|
void setSubloadCallback(SubloadCallback* cb) { _subloadCallback = cb;; }
|
|
|
|
SubloadCallback* getSubloadCallback() { return _subloadCallback.get(); }
|
|
|
|
const SubloadCallback* getSubloadCallback() const { return _subloadCallback.get(); }
|
|
|
|
|
|
/** Set the number of mip map levels the the texture has been created with,
|
|
should only be called within an osg::Texuture::apply() and custom OpenGL texture load.*/
|
|
void setNumMipmapLevels(unsigned int num) const { _numMipmapLevels=num; }
|
|
|
|
/** Get the number of mip map levels the the texture has been created with.*/
|
|
unsigned int getNumMipmapLevels() const { return _numMipmapLevels; }
|
|
|
|
|
|
/** Copy pixels into a 2D texture image.As per glCopyTexImage2D.
|
|
* Creates an OpenGL texture object from the current OpenGL background
|
|
* framebuffer contents at pos \a x, \a y with width \a width and
|
|
* height \a height. \a width and \a height must be a power of two.
|
|
*/
|
|
void copyTexImage2D(State& state, int x, int y, int width, int height );
|
|
|
|
/** Copy a two-dimensional texture subimage. As per glCopyTexSubImage2D.
|
|
* Updates portion of an existing OpenGL texture object from the current OpenGL background
|
|
* framebuffer contents at pos \a x, \a y with width \a width and
|
|
* height \a height. \a width and \a height must be a power of two,
|
|
* and writing into the texture with offset \a xoffset and \a yoffset.
|
|
*/
|
|
void copyTexSubImage2D(State& state, int xoffset, int yoffset, int x, int y, int width, int height );
|
|
|
|
|
|
/** On first apply (unless already compiled), create the minmapped
|
|
* texture and bind it, subsequent apply will simple bind to texture.*/
|
|
virtual void apply(State& state) const;
|
|
|
|
protected :
|
|
|
|
virtual ~Texture2D();
|
|
|
|
virtual void computeInternalFormat() const;
|
|
|
|
|
|
// not ideal that _image is mutable, but its required since
|
|
// Image::ensureDimensionsArePowerOfTwo() can only be called
|
|
// in a valid OpenGL context, a therefore within an Texture::apply
|
|
// which is const...
|
|
ref_ptr<Image> _image;
|
|
|
|
// subloaded images can have different texture and image sizes.
|
|
mutable GLsizei _textureWidth, _textureHeight;
|
|
|
|
// number of mip map levels the the texture has been created with,
|
|
mutable GLsizei _numMipmapLevels;
|
|
|
|
ref_ptr<SubloadCallback> _subloadCallback;
|
|
|
|
typedef buffered_value<unsigned int> ImageModifiedTag;
|
|
mutable ImageModifiedTag _modifiedTag;
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|