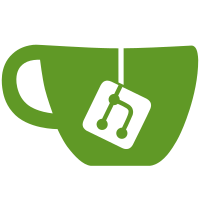
osg::GraphicsContext, in order to give good integration with the application's GUI toolkit. This works really well. However, I need to share OpenGL texture resources with the standard osgViewer GraphicsContext implementations, in particular the PixelBuffers. This is essential for my application to conserve graphics memory on low-end hardware. Currently the standard osg implementations will not share resources with another derived osg::GraphicsContext, other than the pre-defined osgViewer classes e.g. PixelBufferX11 is hardcoded to only share resources with GraphicsWindowX11 and PixelBufferX11 objects, and no other osg::GraphicsContext object. To address this in the cleanest way I could think of, I have moved the OpenGL handle variables for each platform into a small utility class, e.g. GraphicsHandleX11 for unix. Then GraphicsWindowX11, PixelBufferX11 and any other derived osg::GraphicsContext class can inherit from GraphicsHandleX11 to share OpenGL resources. I have updated the X11, Win32 and Carbon implementations to use this. The changes are minor. I haven't touched the Cocoa implmentation as I'm not familiar with it at all and couldn't test it - it will work unchanged. Without this I had some horrible hacks in my application, this greatly simplifies things for me. It also simplifies the osgViewer implementations slightly. Perhaps it may help with other users' desires to share resources with external graphics contexts, as was discussed on the user list recently." Notes from Robert Osfield, adapted Colin's submission to work with the new EGL related changes.
91 lines
2.9 KiB
C++
91 lines
2.9 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2006 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
/* Note, elements of PixelBufferX11 have used Prodcer/RenderSurface_X11.cpp as both
|
|
* a guide to use of X11/GLX and copiying directly in the case of setBorder().
|
|
* These elements are license under OSGPL as above, with Copyright (C) 2001-2004 Don Burns.
|
|
*/
|
|
|
|
#ifndef OSGVIEWER_PIXELBUFFERX11
|
|
#define OSGVIEWER_PIXELBUFFERX11 1
|
|
|
|
#include <osg/GraphicsContext>
|
|
#include <osgViewer/api/X11/GraphicsHandleX11>
|
|
|
|
namespace osgViewer
|
|
{
|
|
|
|
class OSGVIEWER_EXPORT PixelBufferX11 : public osg::GraphicsContext, public osgViewer::GraphicsHandleX11
|
|
{
|
|
public:
|
|
|
|
PixelBufferX11(osg::GraphicsContext::Traits* traits);
|
|
|
|
virtual bool isSameKindAs(const Object* object) const { return dynamic_cast<const PixelBufferX11*>(object)!=0; }
|
|
virtual const char* libraryName() const { return "osgViewer"; }
|
|
virtual const char* className() const { return "PixelBufferX11"; }
|
|
|
|
virtual bool valid() const { return _valid; }
|
|
|
|
/** Realise the GraphicsContext.*/
|
|
virtual bool realizeImplementation();
|
|
|
|
/** Return true if the graphics context has been realised and is ready to use.*/
|
|
virtual bool isRealizedImplementation() const { return _realized; }
|
|
|
|
/** Close the graphics context.*/
|
|
virtual void closeImplementation();
|
|
|
|
/** Make this graphics context current.*/
|
|
virtual bool makeCurrentImplementation();
|
|
|
|
/** Make this graphics context current with specified read context implementation. */
|
|
virtual bool makeContextCurrentImplementation(osg::GraphicsContext* readContext);
|
|
|
|
/** Release the graphics context.*/
|
|
virtual bool releaseContextImplementation();
|
|
|
|
/** Bind the graphics context to associated texture implementation.*/
|
|
virtual void bindPBufferToTextureImplementation(GLenum buffer);
|
|
|
|
/** Swap the front and back buffers.*/
|
|
virtual void swapBuffersImplementation();
|
|
|
|
public:
|
|
|
|
// X11 specific aces functions
|
|
|
|
Pbuffer& getPbuffer() { return _pbuffer; }
|
|
|
|
protected:
|
|
|
|
~PixelBufferX11();
|
|
|
|
bool createVisualInfo();
|
|
|
|
void init();
|
|
|
|
bool _valid;
|
|
Pbuffer _pbuffer;
|
|
XVisualInfo* _visualInfo;
|
|
|
|
bool _initialized;
|
|
bool _realized;
|
|
|
|
bool _useGLX1_3;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|