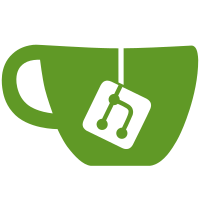
forcing users to use osgDB::readRef*File() methods. The later is preferable as it closes a potential threading bug when using paging databases in conjunction with the osgDB::Registry Object Cache. This threading bug occurs when one thread gets an object from the Cache via an osgDB::read*File() call where only a pointer to the object is passed back, so taking a reference to the object is delayed till it gets reassigned to a ref_ptr<>, but at the same time another thread calls a flush of the Object Cache deleting this object as it's referenceCount is now zero. Using osgDB::readREf*File() makes sure the a ref_ptr<> is passed back and the referenceCount never goes to zero. To ensure the OSG builds when OSG_PROVIDE_READFILE is to OFF the many cases of osgDB::read*File() usage had to be replaced with a ref_ptr<> osgDB::readRef*File() usage. The avoid this change causing lots of other client code to be rewritten to handle the use of ref_ptr<> in place of C pointer I introduced a serious of templte methods in various class to adapt ref_ptr<> to the underly C pointer to be passed to old OSG API's, example of this is found in include/osg/Group: bool addChild(Node* child); // old method which can only be used with a Node* tempalte<class T> bool addChild(const osg::ref_ptr<T>& child) { return addChild(child.get()); } // adapter template method These changes together cover 149 modified files, so it's a large submission. This extent of changes are warrent to make use of the Object Cache and multi-threaded loaded more robust. git-svn-id: http://svn.openscenegraph.org/osg/OpenSceneGraph/trunk@15164 16af8721-9629-0410-8352-f15c8da7e697
97 lines
2.8 KiB
C++
97 lines
2.8 KiB
C++
#include <iostream>
|
|
#include <osg/Geode>
|
|
#include <osg/TexGen>
|
|
#include <osg/Texture2D>
|
|
#include <osg/MatrixTransform>
|
|
#include <osgDB/ReadFile>
|
|
#include <osgViewer/Viewer>
|
|
#include <osg/ShapeDrawable>
|
|
#include <osg/Material>
|
|
|
|
extern osg::Node * CreateSimpleHierarchy( osg::Node * model );
|
|
extern osg::Node * CreateAdvancedHierarchy( osg::Node * model );
|
|
|
|
////////////////////////////////////////////////////////////////////////////////
|
|
osg::Node * CreateGlobe( void )
|
|
{
|
|
// File not found - create textured sphere
|
|
osg::Geode * geode = new osg::Geode;
|
|
osg::ref_ptr<osg::TessellationHints> hints = new osg::TessellationHints;
|
|
hints->setDetailRatio( 0.3 );
|
|
|
|
#if 1
|
|
osg::ref_ptr<osg::ShapeDrawable> shape = new osg::ShapeDrawable
|
|
( new osg::Sphere(osg::Vec3(0.0f, 0.0f, 0.0f), 4.0 ), hints.get() );
|
|
#else
|
|
osg::ref_ptr<osg::ShapeDrawable> shape = new osg::ShapeDrawable
|
|
( new osg::Box( osg::Vec3(-1.0f, -1.0f, -1.0f), 2.0, 2.0, 2.0 ) );
|
|
#endif
|
|
|
|
shape->setColor(osg::Vec4(0.8f, 0.8f, 0.8f, 1.0f));
|
|
|
|
geode->addDrawable( shape.get() );
|
|
|
|
osg::StateSet * stateSet = new osg::StateSet;
|
|
|
|
osg::Texture2D * texture = new osg::Texture2D(
|
|
osgDB::readRefImageFile("Images/land_shallow_topo_2048.jpg")
|
|
);
|
|
|
|
osg::Material * material = new osg::Material;
|
|
|
|
material->setAmbient
|
|
( osg::Material::FRONT_AND_BACK, osg::Vec4( 0.9, 0.9, 0.9, 1.0 ) );
|
|
|
|
material->setDiffuse
|
|
( osg::Material::FRONT_AND_BACK, osg::Vec4( 0.9, 0.9, 0.9, 1.0 ) );
|
|
|
|
#if 1
|
|
material->setSpecular
|
|
( osg::Material::FRONT_AND_BACK, osg::Vec4( 0.7, 0.3, 0.3, 1.0 ) );
|
|
|
|
material->setShininess( osg::Material::FRONT_AND_BACK, 25 );
|
|
|
|
#endif
|
|
|
|
stateSet->setAttributeAndModes( material );
|
|
stateSet->setTextureAttributeAndModes( 0,texture, osg::StateAttribute::ON );
|
|
|
|
geode->setStateSet( stateSet );
|
|
return geode;
|
|
}
|
|
////////////////////////////////////////////////////////////////////////////////
|
|
int main( int argc, char **argv )
|
|
{
|
|
// construct the viewer.
|
|
osg::ArgumentParser arguments( &argc, argv );
|
|
osgViewer::Viewer viewer( arguments );
|
|
|
|
bool useSimpleExample = arguments.read("-s") || arguments.read("--simple") ;
|
|
|
|
osg::ref_ptr<osg::Node> model;
|
|
|
|
if (arguments.argc()>1 && !arguments.isOption(1) )
|
|
{
|
|
std::string filename = arguments[1];
|
|
model = osgDB::readRefNodeFile( filename );
|
|
if ( !model ) {
|
|
osg::notify( osg::NOTICE )
|
|
<< "Error, cannot read " << filename
|
|
<< ". Loading default earth model instead." << std::endl;
|
|
}
|
|
}
|
|
|
|
if( model == NULL )
|
|
model = CreateGlobe( );
|
|
|
|
osg::ref_ptr<osg::Node> node = useSimpleExample ?
|
|
CreateSimpleHierarchy( model.get() ):
|
|
CreateAdvancedHierarchy( model.get() );
|
|
|
|
viewer.setSceneData( node );
|
|
viewer.realize( );
|
|
viewer.run();
|
|
|
|
return 0;
|
|
}
|