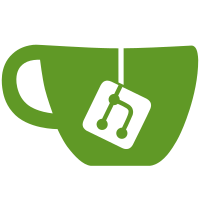
forcing users to use osgDB::readRef*File() methods. The later is preferable as it closes a potential threading bug when using paging databases in conjunction with the osgDB::Registry Object Cache. This threading bug occurs when one thread gets an object from the Cache via an osgDB::read*File() call where only a pointer to the object is passed back, so taking a reference to the object is delayed till it gets reassigned to a ref_ptr<>, but at the same time another thread calls a flush of the Object Cache deleting this object as it's referenceCount is now zero. Using osgDB::readREf*File() makes sure the a ref_ptr<> is passed back and the referenceCount never goes to zero. To ensure the OSG builds when OSG_PROVIDE_READFILE is to OFF the many cases of osgDB::read*File() usage had to be replaced with a ref_ptr<> osgDB::readRef*File() usage. The avoid this change causing lots of other client code to be rewritten to handle the use of ref_ptr<> in place of C pointer I introduced a serious of templte methods in various class to adapt ref_ptr<> to the underly C pointer to be passed to old OSG API's, example of this is found in include/osg/Group: bool addChild(Node* child); // old method which can only be used with a Node* tempalte<class T> bool addChild(const osg::ref_ptr<T>& child) { return addChild(child.get()); } // adapter template method These changes together cover 149 modified files, so it's a large submission. This extent of changes are warrent to make use of the Object Cache and multi-threaded loaded more robust. git-svn-id: http://svn.openscenegraph.org/osg/OpenSceneGraph/trunk@15164 16af8721-9629-0410-8352-f15c8da7e697
121 lines
4.5 KiB
C++
121 lines
4.5 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2006 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSGPARTICLE_PARTICLEEFFECT
|
|
#define OSGPARTICLE_PARTICLEEFFECT
|
|
|
|
#include <osgParticle/Emitter>
|
|
#include <osgParticle/Program>
|
|
|
|
namespace osgParticle
|
|
{
|
|
|
|
class OSGPARTICLE_EXPORT ParticleEffect : public osg::Group
|
|
{
|
|
public:
|
|
|
|
explicit ParticleEffect(bool automaticSetup=true):
|
|
_automaticSetup(automaticSetup),
|
|
_useLocalParticleSystem(true),
|
|
_scale(1.0f),
|
|
_intensity(1.0f),
|
|
_startTime(0.0),
|
|
_emitterDuration(1.0),
|
|
_wind(0.0f,0.0f,0.0f)
|
|
{}
|
|
|
|
ParticleEffect(const ParticleEffect& copy, const osg::CopyOp& copyop = osg::CopyOp::SHALLOW_COPY);
|
|
|
|
|
|
virtual const char* libraryName() const { return "osgParticle"; }
|
|
virtual const char* className() const { return "ParticleEffect"; }
|
|
virtual bool isSameKindAs(const osg::Object* obj) const { return dynamic_cast<const ParticleEffect*>(obj) != 0; }
|
|
virtual void accept(osg::NodeVisitor& nv) { if (nv.validNodeMask(*this)) { nv.pushOntoNodePath(this); nv.apply(*this); nv.popFromNodePath(); } }
|
|
|
|
void setAutomaticSetup(bool flag) { _automaticSetup = flag; }
|
|
bool getAutomaticSetup() const { return _automaticSetup; }
|
|
|
|
void setUseLocalParticleSystem(bool local);
|
|
bool getUseLocalParticleSystem() const { return _useLocalParticleSystem; }
|
|
|
|
void setTextureFileName(const std::string& filename);
|
|
const std::string& getTextureFileName() const { return _textureFileName; }
|
|
|
|
void setDefaultParticleTemplate(const Particle& p);
|
|
const Particle& getDefaultParticleTemplate() const { return _defaultParticleTemplate; }
|
|
|
|
void setPosition(const osg::Vec3& position);
|
|
const osg::Vec3& getPosition() const { return _position; }
|
|
|
|
void setScale(float scale);
|
|
float getScale() const { return _scale; }
|
|
|
|
void setIntensity(float intensity);
|
|
float getIntensity() const { return _intensity; }
|
|
|
|
void setStartTime(double startTime);
|
|
double getStartTime() const { return _startTime; }
|
|
|
|
void setEmitterDuration(double duration);
|
|
double getEmitterDuration() const { return _emitterDuration; }
|
|
|
|
void setParticleDuration(double duration);
|
|
double getParticleDuration() const { return _defaultParticleTemplate.getLifeTime(); }
|
|
|
|
void setWind(const osg::Vec3& wind);
|
|
const osg::Vec3& getWind() const { return _wind; }
|
|
|
|
/// Get whether all particles are dead
|
|
bool areAllParticlesDead() const { return _particleSystem.valid()?_particleSystem->areAllParticlesDead():true; }
|
|
|
|
virtual Emitter* getEmitter() = 0;
|
|
virtual const Emitter* getEmitter() const = 0;
|
|
|
|
virtual Program* getProgram() = 0;
|
|
virtual const Program* getProgram() const = 0;
|
|
|
|
void setParticleSystem(ParticleSystem* ps);
|
|
template<class T> void setParticleSystem(const osg::ref_ptr<T>& ri) { setParticleSystem(ri.get()); }
|
|
|
|
inline ParticleSystem* getParticleSystem() { return _particleSystem.get(); }
|
|
inline const ParticleSystem* getParticleSystem() const { return _particleSystem.get(); }
|
|
|
|
virtual void setDefaults();
|
|
|
|
virtual void setUpEmitterAndProgram() = 0;
|
|
|
|
virtual void buildEffect();
|
|
|
|
protected:
|
|
|
|
virtual ~ParticleEffect() {}
|
|
|
|
bool _automaticSetup;
|
|
|
|
osg::ref_ptr<ParticleSystem> _particleSystem;
|
|
|
|
bool _useLocalParticleSystem;
|
|
std::string _textureFileName;
|
|
Particle _defaultParticleTemplate;
|
|
osg::Vec3 _position;
|
|
float _scale;
|
|
float _intensity;
|
|
double _startTime;
|
|
double _emitterDuration;
|
|
osg::Vec3 _wind;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|