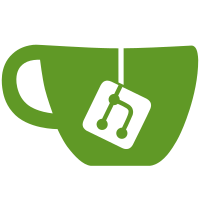
* Test equality of different length strings. * Add tab to json_pack whitespace test. * Test json_sprintf with empty result and invalid UTF. * Test json_get_alloc_funcs with NULL arguments. * Test invalid arguments. * Add test_chaos to test allocation failure code paths. * Remove redundant json_is_string checks from json_string_equal and json_string_copy. Both functions are static and can only be called with a json string. Fixes to issues found by test_chaos: * Fix crash on OOM in pack_unpack.c:read_string(). * Unconditionally free string in string_create upon allocation failure. Update load.c:parse_value() to reflect this. This resolves a leak on allocation failure for pack_unpack.c:pack_string() and value.c:json_sprintf(). Although not visible from CodeCoverage these changes significantly increase branch coverage. Especially in src/value.c where we previously covered 67.4% of branches and now cover 96.3% of branches.
35 lines
841 B
C
35 lines
841 B
C
#include <string.h>
|
|
#include <jansson.h>
|
|
#include "util.h"
|
|
|
|
|
|
static void test_sprintf() {
|
|
json_t *s = json_sprintf("foo bar %d", 42);
|
|
if (!s)
|
|
fail("json_sprintf returned NULL");
|
|
if (!json_is_string(s))
|
|
fail("json_sprintf didn't return a JSON string");
|
|
if (strcmp(json_string_value(s), "foo bar 42"))
|
|
fail("json_sprintf generated an unexpected string");
|
|
|
|
json_decref(s);
|
|
|
|
s = json_sprintf("%s", "");
|
|
if (!s)
|
|
fail("json_sprintf returned NULL");
|
|
if (!json_is_string(s))
|
|
fail("json_sprintf didn't return a JSON string");
|
|
if (json_string_length(s) != 0)
|
|
fail("string is not empty");
|
|
json_decref(s);
|
|
|
|
if (json_sprintf("%s", "\xff\xff"))
|
|
fail("json_sprintf unexpected success with invalid UTF");
|
|
}
|
|
|
|
|
|
static void run_tests()
|
|
{
|
|
test_sprintf();
|
|
}
|