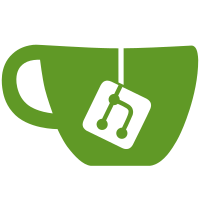
The environment variables that specified the full path the the configuration files for dahdi_span_types and dahdi_span_assignments was documented, but they were not actually set from the environment. Also, the case of the variable was changed to match the one for the directory where the rest of the config files are located. Signed-off-by: Shaun Ruffell <sruffell@digium.com>
269 lines
6.1 KiB
Bash
Executable File
269 lines
6.1 KiB
Bash
Executable File
#! /bin/sh
|
|
#
|
|
# /usr/sbin/dahdi_span_types
|
|
#
|
|
# This script can be used both from udev and
|
|
# from the command line to manage PRI spans
|
|
# type (E1/T1/J1).
|
|
#
|
|
# Span types can be set only *BEFORE* span are assigned.
|
|
#
|
|
# It uses a configuration file: $DAHDICONFDIR/span-types.conf
|
|
# (default DAHDICONFDIR=/etc/dahdi)
|
|
# (the format is documented inside that file)
|
|
#
|
|
# The first argument is an action:
|
|
# "set" - actually write the setting to the driver
|
|
# "list" - human-readable list of E1/T1/J1 types
|
|
# "dumpconfig" - dump current assignments in a /etc/dahdi/span-types.conf
|
|
# compatible format
|
|
#
|
|
# Without further arguments, it operates on all existing spans
|
|
# With one or more sysfs dahdi_devices it is limited to those.
|
|
#
|
|
# We may use alternative "keys" for device matching:
|
|
# * Available keys:
|
|
# - "hwid" - Hardware id attribute from sysfs
|
|
# - "@location" - Location attribute from sysfs (embeded inside '<>')
|
|
# - "/devpath" - The sysfs absolute devpath
|
|
#
|
|
# * During "dumpconfig", for each device we take the first available key:
|
|
# - The preference is: "hwid" or else "@location" or else "/devpath"
|
|
# - This can be overriden via the SPAN_ASSIGNMENTS_KEY environment variable
|
|
# or the '{-k|--key} key' command line option.
|
|
#
|
|
# Command line options:
|
|
# - The '-h|--help' show a usage message.
|
|
# - The '-k <key>|--key <key>' overrides the SPAN_ASSIGNMENTS_KEY environment
|
|
# variable.
|
|
#
|
|
# Examples:
|
|
# dahdi_span_types list
|
|
# dahdi_span_types set # all devices
|
|
# dahdi_span_types set /sys/bus/dahdi_devices/devices/astribanks:xbus-00
|
|
# dahdi_span_types -k location dumpconfig
|
|
#
|
|
|
|
|
|
devbase='/sys/bus/dahdi_devices/devices'
|
|
DAHDICONFDIR="${DAHDICONFDIR:-/etc/dahdi}"
|
|
DAHDISPANTYPESCONF="${DAHDISPANTYPESCONF:-"${DAHDICONFDIR}/span-types.conf"}"
|
|
SPAN_ASSIGNMENTS_KEY=${SPAN_ASSIGNMENTS_KEY:-hwid}
|
|
|
|
usage() {
|
|
echo >&2 "Usage: $0 [options] action [devpath ...]"
|
|
echo >&2 " action:"
|
|
echo >&2 " set - set spans to E1/T1 according to configuration"
|
|
echo >&2 " list - human-readable list of all spans"
|
|
echo >&2 " dumpconfig - dump current state as new configuration"
|
|
echo >&2 ""
|
|
echo >&2 " options:"
|
|
echo >&2 " -h|--help - Show this help"
|
|
echo >&2 " -k|--key <k> - Override prefered key during dumpconfig action"
|
|
exit 1
|
|
}
|
|
|
|
# Parse command line options
|
|
TEMP=`getopt -o hk: --long help,key: -n "$0" -- "$@"`
|
|
if [ $? != 0 ]; then
|
|
echo >&2 "Bad options"
|
|
usage
|
|
fi
|
|
|
|
# Note the quotes around `$TEMP': they are essential!
|
|
eval set -- "$TEMP"
|
|
|
|
while true ; do
|
|
case "$1" in
|
|
-h|--help)
|
|
usage
|
|
;;
|
|
-k|--key)
|
|
SPAN_ASSIGNMENTS_KEY="$2"
|
|
shift
|
|
shift
|
|
;;
|
|
--)
|
|
shift
|
|
break
|
|
;;
|
|
*)
|
|
echo "Internal error!"
|
|
exit 1
|
|
;;
|
|
esac
|
|
done
|
|
|
|
if [ "$#" -eq 0 ]; then
|
|
echo >&2 "Missing action argument"
|
|
usage
|
|
fi
|
|
action="$1"
|
|
shift
|
|
|
|
# Validate SPAN_ASSIGNMENTS_KEY
|
|
case "$SPAN_ASSIGNMENTS_KEY" in
|
|
hwid|location|devpath)
|
|
;;
|
|
*)
|
|
echo >&2 "Bad SPAN_ASSIGNMENTS_KEY='$SPAN_ASSIGNMENTS_KEY' (should be: hwid|location|devpath)"
|
|
usage
|
|
;;
|
|
esac
|
|
|
|
if [ ! -d "$devbase" ]; then
|
|
echo >&2 "$0: Missing '$devbase' (DAHDI driver unloaded?)"
|
|
exit 1
|
|
fi
|
|
|
|
# Use given devices or otherwise, all existing devices
|
|
if [ "$#" -gt 0 ]; then
|
|
DEVICES="$@"
|
|
else
|
|
DEVICES=`echo $devbase/*`
|
|
fi
|
|
|
|
# Beware of special characters in attributes
|
|
attr_clean() {
|
|
cat "$1" | tr -d '\n' | tr '!' '/' | tr -c 'a-zA-Z0-9/:.-' '_'
|
|
}
|
|
|
|
show_spantypes() {
|
|
echo "# PRI span types (E1/T1/J1)"
|
|
for device in $DEVICES
|
|
do
|
|
devpath=`cd "$device" && pwd -P`
|
|
location='@'`attr_clean "$device/location"`
|
|
hardware_id=`attr_clean "$device/hardware_id"`
|
|
cat "$device/spantype" | while read st; do
|
|
case "$st" in
|
|
*:[ETJ]1)
|
|
printf "%-10s %-20s %s\n" \
|
|
"$st" "[$hardware_id]" "$location"
|
|
;;
|
|
esac
|
|
done | sort -n
|
|
done
|
|
}
|
|
|
|
dump_config() {
|
|
echo '#'
|
|
echo "# Autogenerated by $0 on `date`"
|
|
echo "# Map PRI DAHDI devices to span types for E1/T1/J1"
|
|
echo ''
|
|
fmt="%-65s %s\n"
|
|
printf "$fmt" '# @location/hardware_id' 'span_type'
|
|
for device in $DEVICES
|
|
do
|
|
devpath=`cd "$device" && pwd -P`
|
|
location=`attr_clean "$device/location"`
|
|
hardware_id=`attr_clean "$device/hardware_id"`
|
|
if [ "$SPAN_ASSIGNMENTS_KEY" = 'hwid' -a "$hardware_id" != '' ]; then
|
|
id="$hardware_id"
|
|
elif [ "$SPAN_ASSIGNMENTS_KEY" = 'location' -a "$location" != '' ]; then
|
|
id="@$location"
|
|
else
|
|
id="$devpath"
|
|
fi
|
|
echo "# Device: [$hardware_id] @$location $devpath"
|
|
cat "$device/spantype" | while read st; do
|
|
case "$st" in
|
|
*:[ETJ]1)
|
|
printf "$fmt" "$id" "$st"
|
|
;;
|
|
*)
|
|
#echo "# Skipped local span `echo $st | sed 's/:/ -- /'`"
|
|
;;
|
|
esac
|
|
done | sort -n
|
|
#echo ''
|
|
done
|
|
}
|
|
|
|
# Allow comments and empty lines in config file
|
|
filter_conf() {
|
|
sed -e 's/#.*//' -e '/^[ \t]*$/d' "$DAHDISPANTYPESCONF"
|
|
}
|
|
|
|
conf_spans() {
|
|
hardware_id="$1"
|
|
location="$2"
|
|
devpath="$3"
|
|
filter_conf | (
|
|
# Collect device spans
|
|
# in a subshell, so $SPANS is not lost
|
|
SPANS=''
|
|
while read id spans; do
|
|
# GLOBBING
|
|
case "$location" in
|
|
$id)
|
|
#echo >&2 "match($id): $spans"
|
|
SPANS="$SPANS $spans"
|
|
;;
|
|
esac
|
|
case "$hardware_id" in
|
|
$id)
|
|
#echo >&2 "match([$id]): $spans"
|
|
SPANS="$SPANS $spans"
|
|
;;
|
|
esac
|
|
case "$devpath" in
|
|
$id)
|
|
#echo >&2 "match([$id]): $spans"
|
|
SPANS="$SPANS $spans"
|
|
;;
|
|
esac
|
|
done
|
|
echo "$SPANS"
|
|
)
|
|
}
|
|
|
|
device_set_spantype() {
|
|
device="$1"
|
|
attr_file="$device/spantype"
|
|
devpath=`cd "$device" && pwd -P`
|
|
location='@'`attr_clean "$device/location"`
|
|
hardware_id=`attr_clean "$device/hardware_id"`
|
|
spanspecs=`conf_spans "$hardware_id" "$location" "$devpath"`
|
|
#echo >&2 "MATCHED($device): $spanspecs"
|
|
cut -d: -f1 "$attr_file" | while read spanno; do
|
|
for sp in $spanspecs
|
|
do
|
|
s=`echo "$sp" | cut -d: -f1`
|
|
v=`echo "$sp" | cut -d: -f2`
|
|
case "$spanno" in
|
|
$s)
|
|
#echo >&2 "conf($attr_file): $spanno:$v"
|
|
echo "$spanno:$v" > "$attr_file"
|
|
;;
|
|
esac
|
|
done
|
|
done
|
|
}
|
|
|
|
set_spantypes() {
|
|
if [ ! -f "$DAHDISPANTYPESCONF" ]; then
|
|
echo >&2 "$0: Missing configuration '$DAHDISPANTYPESCONF'"
|
|
exit 1
|
|
fi
|
|
for device in $DEVICES
|
|
do
|
|
device_set_spantype "$device"
|
|
done
|
|
}
|
|
|
|
case "$action" in
|
|
list)
|
|
show_spantypes
|
|
;;
|
|
dumpconfig)
|
|
dump_config
|
|
;;
|
|
set)
|
|
set_spantypes
|
|
;;
|
|
*)
|
|
usage
|
|
;;
|
|
esac
|