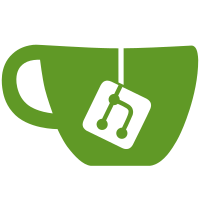
Adds wake lock feature, which is available only for mobile users using browser with support to the given API. When an user using a supported device joins the meeting, a toast is displayed offering to activate the feature. Adds a toggle under settings menu to activate/deactivate it.
134 lines
3.4 KiB
JavaScript
134 lines
3.4 KiB
JavaScript
import React, { Component } from 'react';
|
|
import PropTypes from 'prop-types';
|
|
import { defineMessages, injectIntl } from 'react-intl';
|
|
import { toast } from 'react-toastify';
|
|
import { notify } from '/imports/ui/services/notification';
|
|
import Settings from '/imports/ui/services/settings';
|
|
import Styled from './styles';
|
|
|
|
const intlMessages = defineMessages({
|
|
wakeLockOfferTitle: {
|
|
id: 'app.toast.wakeLock.offerTitle',
|
|
},
|
|
wakeLockOfferAcceptButton: {
|
|
id: 'app.toast.wakeLock.offerAccept',
|
|
},
|
|
wakeLockOfferDeclineButton: {
|
|
id: 'app.toast.wakeLock.offerDecline',
|
|
},
|
|
wakeLockAcquireSuccess: {
|
|
id: 'app.toast.wakeLock.acquireSuccess',
|
|
},
|
|
wakeLockAcquireFailed: {
|
|
id: 'app.toast.wakeLock.acquireFailed',
|
|
},
|
|
});
|
|
|
|
const propTypes = {
|
|
intl: PropTypes.objectOf(Object).isRequired,
|
|
request: PropTypes.func.isRequired,
|
|
release: PropTypes.func.isRequired,
|
|
wakeLockSettings: PropTypes.bool.isRequired,
|
|
};
|
|
|
|
class WakeLock extends Component {
|
|
constructor() {
|
|
super();
|
|
this.notification = null;
|
|
}
|
|
|
|
componentDidMount() {
|
|
const { intl, request } = this.props;
|
|
|
|
const toastProps = {
|
|
closeOnClick: false,
|
|
autoClose: false,
|
|
closeButton: false,
|
|
};
|
|
|
|
const closeNotification = () => {
|
|
toast.dismiss(this.notification);
|
|
this.notification = null;
|
|
};
|
|
|
|
const declineButton = () => (
|
|
<Styled.CloseButton
|
|
type="button"
|
|
onClick={closeNotification}
|
|
>
|
|
{ intl.formatMessage(intlMessages.wakeLockOfferDeclineButton) }
|
|
</Styled.CloseButton>
|
|
);
|
|
|
|
const acceptButton = () => (
|
|
<Styled.AcceptButton
|
|
type="button"
|
|
onClick={async () => {
|
|
closeNotification();
|
|
const success = await request();
|
|
if (success) {
|
|
Settings.application.wakeLockEnabled = true;
|
|
Settings.save();
|
|
}
|
|
this.feedbackToast(success);
|
|
}}
|
|
>
|
|
{ intl.formatMessage(intlMessages.wakeLockOfferAcceptButton) }
|
|
</Styled.AcceptButton>
|
|
);
|
|
|
|
const toastContent = this.getToast('wakeLockOffer', 'wakeLockOfferTitle', acceptButton(), declineButton());
|
|
this.notification = notify(toastContent, 'default', 'lock', toastProps, null, true);
|
|
}
|
|
|
|
getToast(id, title, button1, button2) {
|
|
const { intl } = this.props;
|
|
|
|
return (
|
|
<div id={id}>
|
|
<Styled.Title>
|
|
{ intl.formatMessage(intlMessages[title]) }
|
|
</Styled.Title>
|
|
<Styled.ToastButtons>
|
|
{ button1 }
|
|
{ button2 }
|
|
</Styled.ToastButtons>
|
|
</div>
|
|
);
|
|
}
|
|
|
|
feedbackToast(success) {
|
|
const feedbackToastProps = {
|
|
closeOnClick: true,
|
|
autoClose: true,
|
|
closeButton: false,
|
|
};
|
|
|
|
const feedbackToast = this.getToast(success ? 'wakeLockSuccess' : 'wakeLockFailed',
|
|
success ? 'wakeLockAcquireSuccess' : 'wakeLockAcquireFailed', null, null);
|
|
setTimeout(() => {
|
|
notify(feedbackToast, success ? 'success' : 'error', 'lock', feedbackToastProps, null, true);
|
|
}, 800);
|
|
}
|
|
|
|
render() {
|
|
const { wakeLockSettings, request, release } = this.props;
|
|
if (wakeLockSettings) {
|
|
request().then((success) => {
|
|
if (!success) {
|
|
this.feedbackToast(success);
|
|
Settings.application.wakeLockEnabled = false;
|
|
Settings.save();
|
|
}
|
|
});
|
|
} else {
|
|
release();
|
|
}
|
|
return null;
|
|
}
|
|
}
|
|
|
|
WakeLock.propTypes = propTypes;
|
|
|
|
export default injectIntl(WakeLock);
|