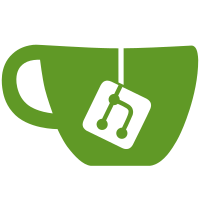
* adds media tests files * fixes the audio test * adds virtualized list test * add default values to .env-template * re-add media folder to ignored list * Revert "adds media tests files" This reverts commitcca05d3513
. * Revert "fixes the audio test" This reverts commit73ef7f69a4
. * fixes the audio test * updates puppeteer from 1.7.0 to 2.0.0 to fix Page.setInterceptFileChooserDialog problems * [WIP]: adding the collection of number of users in DOM and mongo * updates virtualized user list test * browserless as a singleton * adds some console logs * adds logs and code updates * updates code and deleted singleton * deleted the dateObj from Page.getMetrics() * updates .env-template variables * updates the test to return if success or fail * removes debugging comments and updates w/ or w/o browserless page args
37 lines
1.2 KiB
JavaScript
37 lines
1.2 KiB
JavaScript
const ae = require('./elements');
|
|
|
|
async function joinAudio(test) {
|
|
await test.waitForSelector(ae.joinAudio);
|
|
await test.page.evaluate(clickTestElement, ae.joinAudio);
|
|
await test.waitForSelector(ae.listen);
|
|
await test.page.evaluate(clickTestElement, ae.listen);
|
|
await test.waitForSelector(ae.connectingStatus);
|
|
await test.elementRemoved(ae.connectingStatus);
|
|
await test.waitForSelector(ae.leaveAudio);
|
|
const resp = await test.page.evaluate(getTestElement, ae.leaveAudio);
|
|
return resp;
|
|
}
|
|
|
|
async function joinMicrophone(test) {
|
|
await test.waitForSelector(ae.joinAudio);
|
|
await test.page.evaluate(clickTestElement, ae.joinAudio);
|
|
await test.waitForSelector(ae.microphone);
|
|
await test.page.evaluate(clickTestElement, ae.microphone);
|
|
await test.waitForSelector(ae.connectingStatus);
|
|
await test.elementRemoved(ae.connectingStatus);
|
|
await test.waitForSelector(ae.audioAudible);
|
|
const resp = await test.page.evaluate(getTestElement, ae.audioAudible);
|
|
return resp;
|
|
}
|
|
|
|
async function clickTestElement(element) {
|
|
document.querySelectorAll(element)[0].click();
|
|
}
|
|
|
|
async function getTestElement(element) {
|
|
return document.querySelectorAll(element).length >= 1 === true;
|
|
}
|
|
|
|
exports.joinAudio = joinAudio;
|
|
exports.joinMicrophone = joinMicrophone;
|