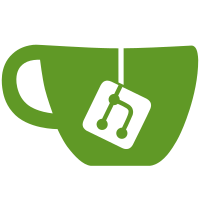
Move all Etherpad's access control from Meteor to a separated [Node application](https://github.com/bigbluebutton/bbb-pads). This new app uses [Etherpad's API](https://etherpad.org/doc/v1.8.4/#index_overview) to create groups and manage session tokens for users to access them. Each group represents one distinct pad at the html5 client. - Removed locked users' access to pads: replaced readOnly pad's access with a new pad's content sharing routine - Pad's access is now controlled by [Etherpad's API](https://etherpad.org/doc/v1.8.4/#index_overview) - Closed captions edited content now reflects at it's live feedback - Improved closed caption's dictation mode live feedback - Moved all Etherpad's API control from Meteor to a separated [app](https://github.com/bigbluebutton/bbb-pads) - Included access control both in akka-apps and bbb-pads
52 lines
1.4 KiB
JavaScript
52 lines
1.4 KiB
JavaScript
import React from 'react';
|
|
import { withTracker } from 'meteor/react-meteor-data';
|
|
import Service from '/imports/ui/components/captions/service';
|
|
import Captions from './component';
|
|
import Auth from '/imports/ui/services/auth';
|
|
import { layoutSelectInput, layoutDispatch } from '../layout/context';
|
|
import { ACTIONS, PANELS } from '/imports/ui/components/layout/enums';
|
|
|
|
const Container = (props) => {
|
|
const cameraDock = layoutSelectInput((i) => i.cameraDock);
|
|
const { isResizing } = cameraDock;
|
|
const layoutContextDispatch = layoutDispatch();
|
|
|
|
const { amIModerator } = props;
|
|
|
|
if (!amIModerator) {
|
|
layoutContextDispatch({
|
|
type: ACTIONS.SET_SIDEBAR_CONTENT_IS_OPEN,
|
|
value: false,
|
|
});
|
|
layoutContextDispatch({
|
|
type: ACTIONS.SET_SIDEBAR_CONTENT_PANEL,
|
|
value: PANELS.NONE,
|
|
});
|
|
return null;
|
|
}
|
|
|
|
return <Captions {...{ layoutContextDispatch, isResizing, ...props }} />;
|
|
};
|
|
|
|
export default withTracker(() => {
|
|
const isRTL = document.documentElement.getAttribute('dir') === 'rtl';
|
|
const {
|
|
locale,
|
|
name,
|
|
ownerId,
|
|
dictating,
|
|
} = Service.getCaptions();
|
|
|
|
return {
|
|
locale,
|
|
name,
|
|
ownerId,
|
|
dictation: Service.canIDictateThisPad(ownerId),
|
|
dictating,
|
|
currentUserId: Auth.userID,
|
|
isRTL,
|
|
hasPermission: Service.hasPermission(),
|
|
amIModerator: Service.amIModerator(),
|
|
};
|
|
})(Container);
|