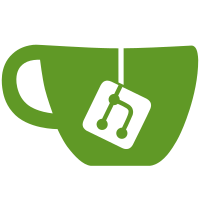
* Fix: allow CORS requests to graphql API In cluster setups the Graphql API endpoints are fetched as a CORS request. We need to allow that. * Fix: Allow CORS requests to ping endpoint In cluster setups the ping is sent directly to the BBB server. So it needs to allow CORS requests for cluster setups. * Fix: construct relative API path for cluster setups * Fix: adjust docs for cluster setup As bbb-html5 client is static, setup instructions for cluster setup have to be changed accordingly. * Fix docs: remove superfluous ```yaml This must have been introduced by accident. --------- Co-authored-by: Daniel Schreiber <daniel.schreiber@hrz.tu-chemnitz.de>
54 lines
1.4 KiB
TypeScript
54 lines
1.4 KiB
TypeScript
import Session from '/imports/ui/services/storage/session';
|
|
import { IndexResponse } from './types';
|
|
|
|
class BBBWebApi {
|
|
private cachePrefix = 'bbbWebApi';
|
|
|
|
private routes = {
|
|
index: {
|
|
// this needs to be a relative path because it may be mounted as a subpath
|
|
// for example in cluster setups
|
|
path: 'bigbluebutton/api',
|
|
cacheKey: `${this.cachePrefix}_index`,
|
|
},
|
|
};
|
|
|
|
private static buildURL(route: string) {
|
|
const pathMatch = window.location.pathname.match('^(.*)/html5client/?$');
|
|
const serverPathPrefix = pathMatch ? `${pathMatch[1]}/` : '';
|
|
const { hostname, protocol } = window.location;
|
|
|
|
return new URL(route, `${protocol}//${hostname}${serverPathPrefix}`);
|
|
}
|
|
|
|
public async index(signal?: AbortSignal): Promise<{
|
|
data: IndexResponse['response'],
|
|
response?: Response,
|
|
}> {
|
|
const cache = Session.getItem(this.routes.index.cacheKey);
|
|
if (cache) {
|
|
return {
|
|
data: cache as IndexResponse['response'],
|
|
};
|
|
}
|
|
|
|
const response = await fetch(BBBWebApi.buildURL(this.routes.index.path), {
|
|
headers: {
|
|
'Content-Type': 'application/json',
|
|
},
|
|
signal,
|
|
});
|
|
|
|
const body: IndexResponse = await response.json();
|
|
Session.setItem(this.routes.index.cacheKey, body.response);
|
|
|
|
return {
|
|
response,
|
|
data: body.response,
|
|
};
|
|
}
|
|
}
|
|
|
|
const BBBWeb = new BBBWebApi();
|
|
export default BBBWeb;
|