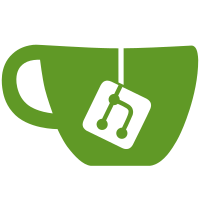
Move all Etherpad's access control from Meteor to a separated [Node application](https://github.com/bigbluebutton/bbb-pads). This new app uses [Etherpad's API](https://etherpad.org/doc/v1.8.4/#index_overview) to create groups and manage session tokens for users to access them. Each group represents one distinct pad at the html5 client. - Removed locked users' access to pads: replaced readOnly pad's access with a new pad's content sharing routine - Pad's access is now controlled by [Etherpad's API](https://etherpad.org/doc/v1.8.4/#index_overview) - Closed captions edited content now reflects at it's live feedback - Improved closed caption's dictation mode live feedback - Moved all Etherpad's API control from Meteor to a separated [app](https://github.com/bigbluebutton/bbb-pads) - Included access control both in akka-apps and bbb-pads
44 lines
1.3 KiB
JavaScript
44 lines
1.3 KiB
JavaScript
import _ from 'lodash';
|
|
import { makeCall } from '/imports/ui/services/api';
|
|
|
|
const DEFAULT_LANGUAGE = 'en-US';
|
|
const THROTTLE_TIMEOUT = 2000;
|
|
|
|
const SpeechRecognitionAPI = window.SpeechRecognition || window.webkitSpeechRecognition;
|
|
|
|
const hasSpeechRecognitionSupport = () => typeof SpeechRecognitionAPI !== 'undefined';
|
|
|
|
const initSpeechRecognition = (locale = DEFAULT_LANGUAGE) => {
|
|
if (hasSpeechRecognitionSupport()) {
|
|
const speechRecognition = new SpeechRecognitionAPI();
|
|
speechRecognition.continuous = true;
|
|
speechRecognition.interimResults = true;
|
|
speechRecognition.lang = locale;
|
|
|
|
return speechRecognition;
|
|
}
|
|
|
|
return null;
|
|
};
|
|
|
|
const pushSpeechTranscript = (locale, transcript, type) => makeCall('pushSpeechTranscript', locale, transcript, type);
|
|
|
|
const throttledTranscriptPush = _.throttle(pushSpeechTranscript, THROTTLE_TIMEOUT, {
|
|
leading: false,
|
|
trailing: true,
|
|
});
|
|
|
|
const pushInterimTranscript = (locale, transcript) => throttledTranscriptPush(locale, transcript, 'interim');
|
|
|
|
const pushFinalTranscript = (locale, transcript) => {
|
|
throttledTranscriptPush.cancel();
|
|
pushSpeechTranscript(locale, transcript, 'final');
|
|
};
|
|
|
|
export default {
|
|
hasSpeechRecognitionSupport,
|
|
initSpeechRecognition,
|
|
pushInterimTranscript,
|
|
pushFinalTranscript,
|
|
};
|