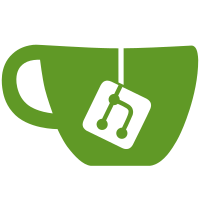
- generate blank slide or thumbnail if conversion fails for that page - if the resulting swf file is greater than 500K, generate a SWF file using a PNG snapshot to make resulting SWF file smaller. This helps to improve performance when rendering the slide on the client Config Changes: /etc/nginx/sites-available/bigbluebutton - set client_max_body_size 20m; // Allow 20Mb file upload - put blank slide and thumbnail in /var/bigbluebutton/blank (see bigbluebutton.properties) git-svn-id: http://bigbluebutton.googlecode.com/svn/trunk@2356 af16638f-c34d-0410-8cfa-b39d5352b314
44 lines
1.3 KiB
Groovy
44 lines
1.3 KiB
Groovy
package org.bigbluebutton.presentation
|
|
|
|
import org.springframework.util.FileCopyUtils
|
|
|
|
class Jpeg2SwfPageConverterTests extends GroovyTestCase {
|
|
|
|
def pageConverter
|
|
def SWFTOOLS_DIR = '/bin'
|
|
def BLANK_SLIDE = '/var/bigbluebutton/blank/blank-slide.swf'
|
|
static final String PRESENTATIONDIR = '/var/bigbluebutton'
|
|
|
|
def conf = "test-conf"
|
|
def rm = "test-room"
|
|
def presName = "thumbs"
|
|
def presDir
|
|
|
|
void setUp() {
|
|
println "Test setup"
|
|
pageConverter = new Jpeg2SwfPageConverter()
|
|
pageConverter.setSwfToolsDir(SWFTOOLS_DIR)
|
|
|
|
presDir = new File("$PRESENTATIONDIR/$conf/$rm/$presName")
|
|
if (presDir.exists()) Util.deleteDirectory(presDir)
|
|
|
|
presDir.mkdirs()
|
|
}
|
|
|
|
void testConvertSlidesSuccessfully() {
|
|
def uploadedFilename = 'sampleslide.jpeg'
|
|
def uploadedFile = new File("test/resources/$uploadedFilename")
|
|
|
|
def uploadedPresentation = new File("$PRESENTATIONDIR/$conf/$rm/$presName/$uploadedFilename")
|
|
int copied = FileCopyUtils.copy(uploadedFile, uploadedPresentation)
|
|
assertTrue(uploadedPresentation.exists())
|
|
|
|
int page = 1
|
|
File output = new File(uploadedPresentation.getParent() + File.separatorChar + "slide-" + page + ".swf")
|
|
|
|
boolean success = pageConverter.convert(uploadedPresentation, output, page)
|
|
assertTrue success
|
|
|
|
}
|
|
}
|