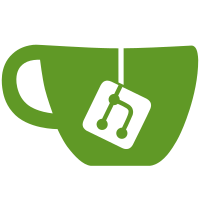
* Refactor: Make all chat area use graphql * Fix: large space between welcome msg and chat list * Fix: missing file * add pending status and fix system messages * Add: mark messages as seen in chat * Refactor: Move char opening logic to inside of chat panel * Refactor message and mark as seen * Add Recharts to package.json and fix miss data * Implements clear-chat function on graphql * Make system message sticky * Add clear message support and fix user is typing * FIx chat unread and scroll not following the tail * Change: make unread messages be marked by message and fix throttle * Don't show restore welcome message when the welcome message isn't set * Fix: scroll not following the tail properly * Fix: previous page last sender not working * Fix: scroll loading all messages * Fix messaga not marked as read --------- Co-authored-by: Gustavo Trott <gustavo@trott.com.br>
65 lines
2.0 KiB
TypeScript
65 lines
2.0 KiB
TypeScript
import React from 'react';
|
|
import ChatHeader from './chat-header/component';
|
|
import { layoutSelect, layoutSelectInput } from '../../layout/context';
|
|
import { Input, Layout } from '../../layout/layoutTypes';
|
|
import Styled from './styles';
|
|
import ChatMessageListContainer from './chat-message-list/component';
|
|
import ChatMessageFormContainer from './chat-message-form/component';
|
|
import ChatTypingIndicatorContainer from './chat-typing-indicator/component';
|
|
import { PANELS, ACTIONS } from '/imports/ui/components/layout/enums';
|
|
import { CircularProgress } from "@mui/material";
|
|
import usePendingChat from '/imports/ui/core/local-states/usePendingChat';
|
|
import useChat from '/imports/ui/core/hooks/useChat';
|
|
import { Chat } from '/imports/ui/Types/chat';
|
|
import { layoutDispatch } from '/imports/ui/components/layout/context';
|
|
interface ChatProps {
|
|
|
|
}
|
|
|
|
const Chat: React.FC<ChatProps> = () => {
|
|
return (
|
|
<Styled.Chat>
|
|
<ChatHeader />
|
|
<ChatMessageListContainer />
|
|
<ChatMessageFormContainer />
|
|
<ChatTypingIndicatorContainer />
|
|
</Styled.Chat>
|
|
);
|
|
};
|
|
|
|
const ChatLoading: React.FC = () => {
|
|
return <Styled.Chat >
|
|
<CircularProgress style={{ alignSelf: 'center' }} />
|
|
</Styled.Chat>;
|
|
};
|
|
|
|
const ChatContainer: React.FC = () => {
|
|
const idChatOpen = layoutSelect((i: Layout) => i.idChatOpen);
|
|
const sidebarContent = layoutSelectInput((i: Input) => i.sidebarContent);
|
|
const layoutContextDispatch = layoutDispatch();
|
|
const chats = useChat((chat) => {
|
|
return {
|
|
chatId: chat.chatId,
|
|
participant: chat.participant,
|
|
};
|
|
}) as Partial<Chat>[];
|
|
|
|
const [pendingChat, setPendingChat] = usePendingChat();
|
|
|
|
if (pendingChat) {
|
|
const chat = chats.find((c) => { return c.participant?.userId === pendingChat });
|
|
if (chat) {
|
|
setPendingChat('');
|
|
layoutContextDispatch({
|
|
type: ACTIONS.SET_ID_CHAT_OPEN,
|
|
value: chat.chatId,
|
|
});
|
|
}
|
|
}
|
|
|
|
if (sidebarContent.sidebarContentPanel !== PANELS.CHAT) return null;
|
|
if (!idChatOpen) return <ChatLoading />;
|
|
return <Chat />;
|
|
};
|
|
|
|
export default ChatContainer; |