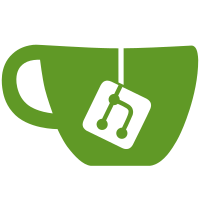
* Refactor: Make all chat area use graphql * Fix: large space between welcome msg and chat list * Fix: missing file * add pending status and fix system messages * Add: mark messages as seen in chat * Refactor: Move char opening logic to inside of chat panel * Refactor message and mark as seen * Add Recharts to package.json and fix miss data * Implements clear-chat function on graphql * Make system message sticky * Add clear message support and fix user is typing * FIx chat unread and scroll not following the tail * Change: make unread messages be marked by message and fix throttle * Don't show restore welcome message when the welcome message isn't set * Fix: scroll not following the tail properly * Fix: previous page last sender not working * Fix: scroll loading all messages * Fix messaga not marked as read --------- Co-authored-by: Gustavo Trott <gustavo@trott.com.br>
34 lines
626 B
TypeScript
34 lines
626 B
TypeScript
import { gql } from '@apollo/client';
|
|
|
|
type chatContent = {
|
|
chatId: string;
|
|
public: boolean;
|
|
participant: {
|
|
name: string;
|
|
};
|
|
};
|
|
|
|
export interface GetChatDataResponse {
|
|
chat: Array<chatContent>;
|
|
}
|
|
|
|
export const GET_CHAT_DATA = gql`
|
|
query GetChatData($chatId: String!) {
|
|
chat(where: { chatId: { _eq: $chatId } }) {
|
|
chatId
|
|
public
|
|
participant {
|
|
name
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const CLOSE_PRIVATE_CHAT_MUTATION = gql`
|
|
mutation UpdateChatUser($chatId: String) {
|
|
update_chat_user(where: { chatId: { _eq: $chatId } }, _set: { visible: false }) {
|
|
affected_rows
|
|
}
|
|
}
|
|
`;
|