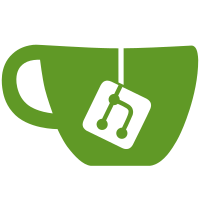
* [plugin-sdk-refactor-organization] - rearrange of files and folders (WIP) * [plugin-sdk-refactor-organization ] - final touches * [plugin-sdk-refactor-organization ] - fix * [plugin-sdk-refactor-organization ] - update SDK
50 lines
1.3 KiB
JavaScript
Executable File
50 lines
1.3 KiB
JavaScript
Executable File
import React from 'react';
|
|
import { useContext } from 'react';
|
|
import { withTracker } from 'meteor/react-meteor-data';
|
|
import { injectIntl } from 'react-intl';
|
|
import JoinVideoButton from './component';
|
|
import VideoService from '../service';
|
|
import {
|
|
updateSettings,
|
|
} from '/imports/ui/components/settings/service';
|
|
import { PluginsContext } from '/imports/ui/components/components-data/plugin-context/context';
|
|
|
|
const JoinVideoOptionsContainer = (props) => {
|
|
const {
|
|
updateSettings,
|
|
hasVideoStream,
|
|
disableReason,
|
|
status,
|
|
intl,
|
|
...restProps
|
|
} = props;
|
|
|
|
const {
|
|
pluginsExtensibleAreasAggregatedState,
|
|
} = useContext(PluginsContext);
|
|
let cameraSettingsDropdownItems = [];
|
|
if (pluginsExtensibleAreasAggregatedState.cameraSettingsDropdownItems) {
|
|
cameraSettingsDropdownItems = [
|
|
...pluginsExtensibleAreasAggregatedState.cameraSettingsDropdownItems,
|
|
];
|
|
}
|
|
return (
|
|
<JoinVideoButton {...{
|
|
cameraSettingsDropdownItems,
|
|
hasVideoStream,
|
|
updateSettings,
|
|
disableReason,
|
|
status,
|
|
...restProps,
|
|
}}
|
|
/>
|
|
);
|
|
};
|
|
|
|
export default injectIntl(withTracker(() => ({
|
|
hasVideoStream: VideoService.hasVideoStream(),
|
|
updateSettings,
|
|
disableReason: VideoService.disableReason(),
|
|
status: VideoService.getStatus(),
|
|
}))(JoinVideoOptionsContainer));
|