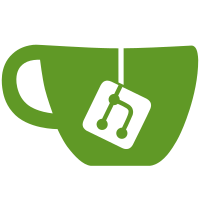
Turns the screenshare component into a generic component, so that it can be used both for screenshare and camera as content fetures. Also changes specific locales and icons for the camera as content feature.
85 lines
2.9 KiB
JavaScript
Executable File
85 lines
2.9 KiB
JavaScript
Executable File
import React from 'react';
|
|
import PropTypes from 'prop-types';
|
|
import { defineMessages, injectIntl } from 'react-intl';
|
|
import Button from '/imports/ui/components/common/button/component';
|
|
|
|
|
|
const propTypes = {
|
|
intl: PropTypes.shape({
|
|
formatMessage: PropTypes.func.isRequired,
|
|
}).isRequired,
|
|
setPresentationIsOpen: PropTypes.func.isRequired,
|
|
};
|
|
|
|
const intlMessages = defineMessages({
|
|
minimizePresentationLabel: {
|
|
id: 'app.actionsBar.actionsDropdown.minimizePresentationLabel',
|
|
description: '',
|
|
},
|
|
minimizePresentationDesc: {
|
|
id: 'app.actionsBar.actionsDropdown.restorePresentationDesc',
|
|
description: '',
|
|
},
|
|
restorePresentationLabel: {
|
|
id: 'app.actionsBar.actionsDropdown.restorePresentationLabel',
|
|
description: 'Restore Presentation option label',
|
|
},
|
|
restorePresentationDesc: {
|
|
id: 'app.actionsBar.actionsDropdown.restorePresentationDesc',
|
|
description: 'button to restore presentation after it has been closed',
|
|
},
|
|
});
|
|
|
|
const PresentationOptionsContainer = ({
|
|
intl,
|
|
presentationIsOpen,
|
|
setPresentationIsOpen,
|
|
layoutContextDispatch,
|
|
hasPresentation,
|
|
hasExternalVideo,
|
|
hasScreenshare,
|
|
hasPinnedSharedNotes,
|
|
hasGenericContent,
|
|
hasCameraAsContent,
|
|
}) => {
|
|
let buttonType = 'presentation';
|
|
if (hasExternalVideo) {
|
|
// hack until we have an external-video icon
|
|
buttonType = 'external-video';
|
|
} else if (hasScreenshare) {
|
|
buttonType = 'desktop';
|
|
} else if (hasCameraAsContent) {
|
|
buttonType = 'video';
|
|
}
|
|
|
|
const isThereCurrentPresentation = hasExternalVideo || hasScreenshare
|
|
|| hasPresentation || hasPinnedSharedNotes
|
|
|| hasGenericContent || hasCameraAsContent;
|
|
return (
|
|
<Button
|
|
icon={`${buttonType}${!presentationIsOpen ? '_off' : ''}`}
|
|
label={intl.formatMessage(!presentationIsOpen ? intlMessages.restorePresentationLabel : intlMessages.minimizePresentationLabel)}
|
|
aria-label={intl.formatMessage(!presentationIsOpen ? intlMessages.restorePresentationLabel : intlMessages.minimizePresentationLabel)}
|
|
aria-describedby={intl.formatMessage(!presentationIsOpen ? intlMessages.restorePresentationDesc : intlMessages.minimizePresentationDesc)}
|
|
description={intl.formatMessage(!presentationIsOpen ? intlMessages.restorePresentationDesc : intlMessages.minimizePresentationDesc)}
|
|
color={presentationIsOpen ? "primary" : "default"}
|
|
hideLabel
|
|
circle
|
|
size="lg"
|
|
onClick={() => {
|
|
setPresentationIsOpen(layoutContextDispatch, !presentationIsOpen);
|
|
if (!hasExternalVideo && !hasScreenshare && !hasPinnedSharedNotes) {
|
|
Session.set('presentationLastState', !presentationIsOpen);
|
|
}
|
|
}}
|
|
id="restore-presentation"
|
|
ghost={!presentationIsOpen}
|
|
disabled={!isThereCurrentPresentation}
|
|
data-test={!presentationIsOpen ? 'restorePresentation' : 'minimizePresentation'}
|
|
/>
|
|
);
|
|
};
|
|
|
|
PresentationOptionsContainer.propTypes = propTypes;
|
|
export default injectIntl(PresentationOptionsContainer);
|