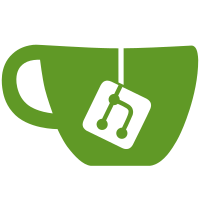
In some cases, `getVoices` returns an empty array even if the browser's vendor has full support for speech synthesis. Add a trigger call to initiate the voices fetching process. As drafted, `getVoices` can be an asynchronous call and monitoring it depends on the support of a `voiceschanged` event. Although many of the main vendors support voices, this event is not (yet) by Safari. https://wicg.github.io/speech-api/#dom-speechsynthesis-getvoices https://wicg.github.io/speech-api/#eventdef-speechsynthesis-voiceschanged https://developer.mozilla.org/en-US/docs/Web/API/SpeechSynthesis/voiceschanged_event
88 lines
2.0 KiB
JavaScript
88 lines
2.0 KiB
JavaScript
import React from 'react';
|
|
import PropTypes from 'prop-types';
|
|
import { defineMessages, injectIntl } from 'react-intl';
|
|
import SpeechService from '/imports/ui/components/audio/captions/speech/service';
|
|
|
|
const intlMessages = defineMessages({
|
|
title: {
|
|
id: 'app.audio.captions.speech.title',
|
|
description: 'Audio speech recognition title',
|
|
},
|
|
disabled: {
|
|
id: 'app.audio.captions.speech.disabled',
|
|
description: 'Audio speech recognition disabled',
|
|
},
|
|
'en-US': {
|
|
id: 'app.audio.captions.select.en-US',
|
|
description: 'Audio speech recognition english language',
|
|
},
|
|
'es-ES': {
|
|
id: 'app.audio.captions.select.es-ES',
|
|
description: 'Audio speech recognition spanish language',
|
|
},
|
|
'pt-BR': {
|
|
id: 'app.audio.captions.select.pt-BR',
|
|
description: 'Audio speech recognition portuguese language',
|
|
},
|
|
});
|
|
|
|
const Select = ({
|
|
intl,
|
|
enabled,
|
|
locale,
|
|
voices,
|
|
}) => {
|
|
if (!enabled) return null;
|
|
|
|
if (SpeechService.useDefault()) return null;
|
|
|
|
if (voices.length === 0) return null;
|
|
|
|
const onChange = (e) => {
|
|
const { value } = e.target;
|
|
SpeechService.setSpeechLocale(value);
|
|
};
|
|
|
|
return (
|
|
<div style={{ padding: '1rem 0' }}>
|
|
<label
|
|
htmlFor="speechSelect"
|
|
style={{ padding: '0 .5rem' }}
|
|
>
|
|
{intl.formatMessage(intlMessages.title)}
|
|
</label>
|
|
<select
|
|
id="speechSelect"
|
|
onChange={onChange}
|
|
value={locale}
|
|
>
|
|
<option
|
|
key="disabled"
|
|
value=""
|
|
>
|
|
{intl.formatMessage(intlMessages.disabled)}
|
|
</option>
|
|
{voices.map((v) => (
|
|
<option
|
|
key={v}
|
|
value={v}
|
|
>
|
|
{intl.formatMessage(intlMessages[v])}
|
|
</option>
|
|
))}
|
|
</select>
|
|
</div>
|
|
);
|
|
};
|
|
|
|
Select.propTypes = {
|
|
enabled: PropTypes.bool.isRequired,
|
|
locale: PropTypes.string.isRequired,
|
|
voices: PropTypes.arrayOf(PropTypes.string).isRequired,
|
|
intl: PropTypes.shape({
|
|
formatMessage: PropTypes.func.isRequired,
|
|
}).isRequired,
|
|
};
|
|
|
|
export default injectIntl(Select);
|