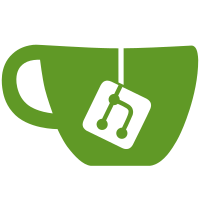
Firefox doesn't fire the ended evt/onended callback for live MediaStreamTrack(s). We rely on that event. Manually emit the ended event which works with the onended callback when a track is stopped
30 lines
673 B
JavaScript
30 lines
673 B
JavaScript
const stopTrack = (track) => {
|
|
if (track && typeof track.stop === 'function' && track.readyState !== 'ended') {
|
|
track.stop();
|
|
// Manually emit the event as a safeguard; Firefox doesn't fire it when it
|
|
// should with live MediaStreamTracks...
|
|
const trackStoppedEvt = new MediaStreamTrackEvent('ended', { track });
|
|
track.dispatchEvent(trackStoppedEvt);
|
|
}
|
|
};
|
|
|
|
const stopStream = (stream) => {
|
|
stream.getTracks().forEach(stopTrack);
|
|
};
|
|
|
|
const silentConsole = {
|
|
log: () => {},
|
|
info: () => {},
|
|
error: () => {},
|
|
warn: () => {},
|
|
debug: () => {},
|
|
trace: () => {},
|
|
assert: () => {},
|
|
};
|
|
|
|
export {
|
|
stopStream,
|
|
stopTrack,
|
|
silentConsole,
|
|
};
|