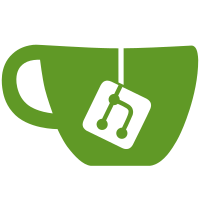
* updating old tests + collecting more snapshots [WIP] * updates old test suites and collects more visual regressions screenshots * remove snapshots and their collection temporary * run tests from packages.json * update test execution command/export constants from .env to core/constants.js * update tests/puppeteer/README.md file * update LOOP_INTERVAL variable call and assign timeouts to the webcam share spec * redefine waitForSelector func in page.js, update chat test suite specs and add poll chat message test spec * Merge remote-tracking branch 'upstream/develop' into updating-old-tests-visual-with-visual-regressions * update webcam test specs collecting videoPreviewTimeout and use it to wait for videoPreview selector * update custom parameters test suite * update breakout test suite * update webcam layout test suite * update multiusers test suite * update notifications test suite * update presentation test suite * whiteboard test suite * screenshare test suite * update sharednotes test suite * user ELEMENT_WAIT_TIME variable from timeouts constants.js * list TEST CONSTANTS by category * add poll test suite and assigns the right unassigned timeouts * set test pages to headless
48 lines
1.6 KiB
JavaScript
48 lines
1.6 KiB
JavaScript
// Test: Cleaning a chat message
|
|
|
|
const Page = require('../core/page');
|
|
const e = require('./elements');
|
|
const util = require('./util');
|
|
const { chatPushAlerts } = require('../notifications/elements');
|
|
|
|
class Clear extends Page {
|
|
constructor() {
|
|
super('chat-clear');
|
|
}
|
|
|
|
async test(testName) {
|
|
await util.openChat(this);
|
|
if (process.env.GENERATE_EVIDENCES === 'true') {
|
|
await this.screenshot(`${testName}`, `01-before-chat-message-send-[${testName}]`);
|
|
}
|
|
// sending a message
|
|
await this.type(e.chatBox, e.message);
|
|
await this.click(e.sendButton, true);
|
|
|
|
if (process.env.GENERATE_EVIDENCES === 'true') {
|
|
await this.screenshot(`${testName}`, `02-after-chat-message-send-[${testName}]`);
|
|
}
|
|
|
|
const chat0 = await this.page.evaluate(() => document.querySelectorAll('p[data-test="chatClearMessageText"]').length === 0);
|
|
|
|
// clear
|
|
await this.click(e.chatOptions, true);
|
|
if (process.env.GENERATE_EVIDENCES === 'true') {
|
|
await this.screenshot(`${testName}`, `03-chat-options-clicked-[${testName}]`);
|
|
}
|
|
await this.click(e.chatClear, true);
|
|
await this.page.waitForFunction(
|
|
'document.querySelector("body").innerText.includes("The public chat history was cleared by a moderator")',
|
|
);
|
|
if (process.env.GENERATE_EVIDENCES === 'true') {
|
|
await this.screenshot(`${testName}`, `04-chat-cleared-[${testName}]`);
|
|
}
|
|
|
|
const chat1 = await this.page.evaluate(() => document.querySelectorAll('p[data-test="chatClearMessageText"]').length === 1);
|
|
|
|
return chat0 === chat1;
|
|
}
|
|
}
|
|
|
|
module.exports = exports = Clear;
|