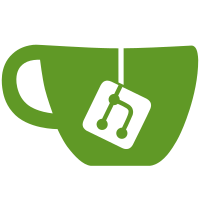
Some browsers seem to (occasionally) not return the getUserMedia promise call and the user gets stuck in this state unable to share her/his webcam. Since enumerateDevices still works even on a gUM rejection this includes a racing timeout that skips gUM. Configured at settings `gUMTimeout`. Reproduced with Windows 10 Chrome 87.
31 lines
620 B
JavaScript
Executable File
31 lines
620 B
JavaScript
Executable File
const promiseTimeout = (ms, promise) => {
|
|
const timeout = new Promise((resolve, reject) => {
|
|
const id = setTimeout(() => {
|
|
clearTimeout(id);
|
|
|
|
const error = {
|
|
name: 'TimeoutError',
|
|
message: 'Promise did not return',
|
|
};
|
|
|
|
reject(error);
|
|
}, ms);
|
|
});
|
|
|
|
return Promise.race([
|
|
promise,
|
|
timeout,
|
|
]);
|
|
};
|
|
|
|
export default {
|
|
promiseTimeout,
|
|
changeWebcam: (deviceId) => {
|
|
Session.set('WebcamDeviceId', deviceId);
|
|
},
|
|
webcamDeviceId: () => Session.get('WebcamDeviceId'),
|
|
changeProfile: (profileId) => {
|
|
Session.set('WebcamProfileId', profileId);
|
|
},
|
|
};
|