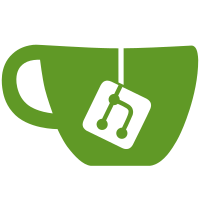
- Poll result as text shape in the lower right-hand - Include all tldraw shape bounds as size in akka (to help Daniel rendering in pdf export) - Default/initital zoom will be centered in the slide and maximize the size according to the presentation area - Also limited the max zoom out to be the one that fits the slide, to be similar as we had before
37 lines
1.5 KiB
JavaScript
37 lines
1.5 KiB
JavaScript
import { withTracker } from "meteor/react-meteor-data";
|
|
import Service from "./service";
|
|
import Whiteboard from "./component";
|
|
import React, { useContext } from "react";
|
|
import { UsersContext } from "../components-data/users-context/context";
|
|
import Auth from "/imports/ui/services/auth";
|
|
import PresentationToolbarService from '../presentation/presentation-toolbar/service';
|
|
|
|
const WhiteboardContainer = (props) => {
|
|
const usingUsersContext = useContext(UsersContext);
|
|
const { users } = usingUsersContext;
|
|
const currentUser = users[Auth.meetingID][Auth.userID];
|
|
const isPresenter = currentUser.presenter;
|
|
return <Whiteboard {...{isPresenter, currentUser}} {...props} meetingId={Auth.meetingID} />
|
|
};
|
|
|
|
export default withTracker(({ whiteboardId, curPageId, intl }) => {
|
|
const shapes = Service.getShapes(whiteboardId, curPageId, intl);
|
|
const assets = Service.getAssets();
|
|
const curPres = Service.getCurrentPres();
|
|
|
|
return {
|
|
initDefaultPages: Service.initDefaultPages,
|
|
persistShape: Service.persistShape,
|
|
persistAsset: Service.persistAsset,
|
|
isMultiUserActive: Service.isMultiUserActive,
|
|
hasMultiUserAccess: Service.hasMultiUserAccess,
|
|
changeCurrentSlide: Service.changeCurrentSlide,
|
|
shapes: shapes,
|
|
assets: assets,
|
|
curPres,
|
|
removeShapes: Service.removeShapes,
|
|
zoomSlide: PresentationToolbarService.zoomSlide,
|
|
skipToSlide: PresentationToolbarService.skipToSlide,
|
|
};
|
|
})(WhiteboardContainer);
|